NEB year 2064 computer science questions
old syllabus:
----------------------------------------------------------
Group 'A' (Long Answer Questions)
Attempt any two of the following questions:[2x20 = 40]
1.
a) Differentiate between break and continue statements with examples. Write a program to print first 10 terms of any series using 'for' loop.[2+3]
Ans:-
Differences are given below:-
break | continue | |
1 | It terminates the execution. | It takes the iteration to next level(skipping). |
2 | It can be used in loop and switch. | It can be used in switch |
3 | It uses keyword ‘break’. | It uses keyword ‘continue’. |
4 | Its syntax is break; | Its syntax is continue; |
5 | Example is For(i=1;i<=4;i++) { If(i<=2) Printf(“%d\n”,i); Break; } } | Example is For(i=1;i<=4;i++) { If(i<=2) Printf(“%d\n”,i); continue; } } |
output is:1 | output is:1,2 |
Second part:-
/*program to print first 10 terms of any series using 'for' loop*/
#include<stdio.h>
int main()
{
int k;
for(k=1;k<=10;k++)
{
printf("%d,",k);
}
return 0;
}
b) What is an operator? Explain assignment, Ternary, and comma operators with examples.[2+3]
Ans:-
operator:-
It is a symbol or a character used in programming. It is used to carry out some operation.
for example: +,-,= etcvariable = expression
Example:
x = a + b
Where x is a variable and a+b is an expression. Here the value of a + b is evaluated and substituted to the variable x.
Ternary operator is also known as a conditional operator as it checks the condition to make decisions. It uses two symbols: the question mark (?) and the colon (:) but not together as In >= ,<= etc. This is the only operator used in C that takes three operands so it is named as ternary operator.
Syntax:
(condition)?statement1:statement2
In this operator, the first condition is checked and if it is found true then statement1 will be executed otherwise statement2 will be executed which is similar to the working style of if-else statement.
Example
a=10;
b=15;
x=(a>b) ? a : b;
Here x will be assigned to the value of b. The condition follows that the expression is false therefore b is assigned to x.
The comma operator can be used to link related expressions together. Comma-linked lists of expressions are evaluated left to right and value of right most expression is the value of the combined expression.
Example
value = (x = 10, y = 5, x + y);
First assigns 10 to x and 5 to y and finally assigns 15 to value. Since comma has the lowest precedence in operators the parenthesis is necessary.
c)Write a program to read a data file.[5]
Ans:-
#include<stdio.h>
int main()
{
FILE *p;
char name[100];
int stdno;
int eng,phy,maths,chem,csc;
p=fopen("student.dat","r");
printf("data are\n");
while((fscanf(p,"%s%d%d%d%d%d%d",name,&stdno,&eng,&phy,&maths,&chem,&csc))!=EOF)
{
printf("name=%s,stdno=%d, english=%d,physics=%d,maths=%d,chemistry=%d ,csc=%d \n",name,stdno,eng,phy,maths,chem,csc);
}
fclose(p);
return 0;
}
d) Write the advantage of function. Write a recursive function to calculate the factorial of any integer number. [5]
Ans:-
Advantages of function:-
Definition: A self –contained block of code that performs a particular task is called Function.
1. It facilitates top down modular programming.
2. The length of a source program can be reduced by using functions at appropriate places.
3. It is easy to locate and isolate a faulty function for further investigations.
4. A function may be used by many other programs.
#include <stdio.h>
#include <stdlib.h>
int recur(int n);
int main()
{
int n;
printf("ente a number\n");
scanf("%d",&n);
printf("the factorial value is\n");
printf("%d",recur(n));
getch();
return 0;
}
int recur(int n)
{
if(n<=0)
{
return 1;
}
else
{
return n*recur(n-1);
}
}
2.
a) Describe the importance of any array. Write a program to store ten differentconstant variables in an array and print out the greatest number. [3+7]
Ans:-
Array:-- handle similar types of data in a program.
- solve problems like sorting, searching, indexing etc.
- to matrix, therefore it is easy for solving matrix related problems. And
- Graphic is an array of pixels, so graphics manipulation can be easily done using an array.
b)Write a program that reads different names and addresses into the computer and sorts the names into alphabetical order using structure variables. [10]
Ans:-
/*
C program to input name and adress n number of people and print them
in alphabetical order and on the basis of name.
*/
#include <stdio.h>
#include<string.h>
struct shorting
{
char name[100];
char address[100];
}var[200],var1;
int main()
{
int i,j,n;
printf("enter total size of data\n");
scanf("%d",&n);
printf("enter name and address\n");
for(i=0;i<n;i++)
{
scanf("%s",var[i].name);
scanf("%s",var[i].address);
}
for(i=0;i<n;i++)
{
for(j=i+1;j<n;j++)
{
if((strcmp(var[i].name,var[j].name))>0)
{
var1=var[i];
var[i]=var[j];
var[j]=var1;
}
}
}
for(i=0;i<n;i++)
{
printf("name=%s address=%s\n",var[i].name,var[i].address);
}
return 0;
}
3.
a) Write a program to delete and rename data file using remove and rename command.[10]
Ans:-
remove():this function is used to delete data file from the system.
b) Write a program to count the number of vowels and consonants in a given text. [10]
Ans:-
/*to find total vowels and consonant present in a string */ #include <stdio.h> #include<string.h> int main() { char str[50]; int i,count,count1; count=count1=0; printf("Enter a string : "); scanf("%[^\n]",str); //strlwr(str); for(i=0;str[i]!='\0';i++) { if(str[i]>=97 && str[i]<=122) { if(str[i]!='a' && str[i]!='e' && str[i]!='i' && str[i]!='o' && str[i]!='u' ) { count++; } else { count1++; } } } printf("total vowels= %d and consonants=%d",count1,count); return 0; } Group 'B' (Short Answer Questions)
Attempt any five questions:[5x7= 35]
4. Differentiate between database and database management system. Explain the top down methodology of database design.[3+4]
Ans:-
Database:-A database is a collection of data organized in a manner that allows access, retrieval, and use of that data. Data is a collection of unprocessed items, which can include text, numbers, images, audio, and video. Information is processed data; that is, it is organized, meaningful, and useful.
DBMS
With database software, often called a database management system (DBMS), users create a computerized database; add, modify, and delete data in the database; sort and retrieve data from the database; and create forms and reports from the data in the database. Database software includes many powerful features.
This methodology carries following properties.
- Breaks the massive problem into smaller subproblems.
- Submodules are solitarily analysed
- communication is not required in the top-down approach.
- Contain redundant information.
- Structure/procedural oriented programming languages (i.e. C) follows the top-down approach.
- It is mainly used in Module documentation, test case creation, code implementation and debugging.
5. What do you understand by AI? How it affects the society? Explain the term polymorphism and inheritance in terms of OOPs.[1+2+4]
Ans:-
AI:-
Artificial intelligence is where machines can learn and make decisions similarly to humans. There are many types of artificial intelligence including machine learning, where instead of being programmed what to think, machines can observe, analyse and learn from data and mistakes just like our human brains can. This technology is influencing consumer products and has led to significant breakthroughs in healthcare and physics as well as altered industries as diverse as manufacturing, finance and retail. In part due to the tremendous amount of data we generate every day and the computing power available, artificial intelligence has exploded in recent years. We might still be years away from generalised AI—when a machine can do anything a human brain can do—, but AI in its current form is still an essential part of our world.
- Artificial Intelligence Influences Business
- Life-Saving AI
- Entertaining AI
- e-mail communications
- self driving cars and buses
- Navigation
- web searchingetc
Second part:-
6. Who is system analyst? Explain the different stages of system development life cycle.[2+5]
Ans:-
SDLC is a structure or a process to develop a software followed by a development team within the software organization. It consists of a detailed plan describing how to develop, maintain and replace specific software. SDLC is also known as information systems development or application development. It is life cycle defining a methodology for improving the quality of software and the overall development process.
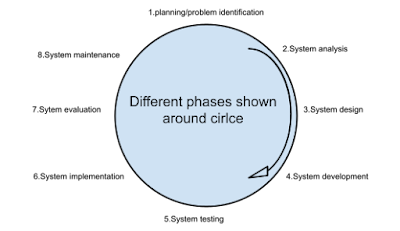
The first step is problem definition(study). The intent is to identify the problem, determine its cause, and outline a strategy for solving it. It defines what ,when who and how project will be carried out.
As we know everything starts with a concept. It could be a concept of someone, or everyone. However, there are those that do not start out with a concept but with a question, “What do you want?” and "really, is there a problem?" They ask thousands of people in a certain community or age group to know what they want and decide to create an answer. But it all goes back to planning and conceptualization.
In this phase the user identifies the need for a new or changes in old or an improved system in large organizations. This identification may be a part of system planning process. Information requirements of the organization as a whole are examined, and projects to meet these requirements are proactively identified.
We can apply techniques like:
1) Collecting data by about system by measuring things, counting things, survey or interview with workers, management, customers, and corporate partners to discover what these people know.
2) observing the processes in action to see where problems lie and improvements can be made in workflow.
3)Research similar systems elsewhere to see how similar problems have been addressed, test the existing system, study the workers in the organization and list the types of information the system needs to produce.
2) Getting the idea about context of problem
3) The processes - you need to know how data is transformed into information.
Like data structures, storage, constraints that must be put on the solution (e.g. operating system that is used, hardware power, minimum speed required etc, what strategy will be best to manage the solution etc.
7. What is computer animation? How is it used in film making industry?[2+5]
Ans:-
Animation:-
Computer animation is the art of creating moving images via the use of computers. It is a subfield of computer graphics and animation. ... Computer animation is essentially a digital successor to the art of stop motion animation of 3D models and frame-by-frame animation of 2D illustrations.8. Explain the benefits of centralized database. What are the major responsibilities of a database Administrator? Explain in detail.[3+4]
Ans:-
Centralized database:-
A centralized database is stored at a single location such as a mainframe computer. It is maintained and modified from that location only and usually accessed using an internet connection such as a LAN or WAN. The centralized database is used by organisations such as colleges, companies, banks etc.Benefits of centralized database:-
Some advantages of Centralized Database Management System are −
- The data integrity is maximised as the whole database is stored at a single physical location. This means that it is easier to coordinate the data and it is as accurate and consistent as possible.
- The data redundancy is minimal in the centralised database. All the data is stored together and not scattered across different locations. So, it is easier to make sure there is no redundant data available.
- Since all the data is in one place, there can be stronger security measures around it. So, the centralised database is much more secure.
- Data is easily portable because it is stored at the same place.
- The centralized database is cheaper than other types of databases as it requires less power and maintenance.
9. What is feasibility study? Explain different levels of feasibility study?[2+5]
Ans:-
It has following types.
10. What is networking? Distinguish between star topology and ring topology of networking principles with the help of clean diagram.[2+5]
Ans:-
Networking:-
A computer network, often simply referred to as a network, is a collection of computers and devices connected by communications channels that facilitates communications among users and allows users to share resources with other users. Networks may be classified according to a wide variety of characteristics.
Purpose of networking:-
Networking for communication medium (have communication among people;internal or external)
Resource sharing (files, printers, hard drives, cd-rom/)
Higher reliability ( to support a computer by another if that computer is down)
star topology:
A star topology is designed with each node (file server, workstations, and peripherals) connected directly to a central network hub, switch, or concentrator (See fig. 2).Data on a star network passes through the hub, switch, or concentrator before continuing to its destination. The hub, switch, or concentrator manages and controls all functions of the network.
It also acts as a repeater for the data flow. This configuration is common with twisted pair cable; however, it can also be used with coaxial cable or fiber optic cable.
Fig. 2. Star topology
Advantages of a Star Topology
Easy to install and wire.
No disruptions to the network when connecting or removing devices.
Easy to detect faults and to remove parts.
Disadvantages of a Star Topology
Requires more cable length than a linear topology.
If the hub, switch, or concentrator fails, nodes attached are disabled.
- Reduced chances of data collision as each node release a data packet after receiving the token.
- Token passing makes ring topology perform better than bus topology under heavy traffic.
- No need of server to control connectivity among the nodes.10. Describe the wireless network system. List out deices and equipment necessary for Wi-Fi network. 3+2
11. What do your mean by data security? Explain briefly the types of security methods normally adopted in computerized environment.[2+5]
Ans:-
Data security:-
It simply says protection of data /information contained in database against unauthorized access, modification or destruction. The main condition for database security is to have “database integrity’ which says about mechanism to keep data in consistent form.
We can apply following methods to secure our data.
Access control. This refers to controlling which users have access to the network or especially sensitive sections of the network.
Antivirus and anti-malware software. Malware, or “malicious software,” is a common form of cyberattack that comes in many different shapes and sizes.
Behavioral analytics.
In order to identify abnormal behavior, security support personnel need to establish a baseline of what constitutes normal behavior for a given customer’s users,
Data loss prevention.
- Data loss prevention (DLP) technologies are those that prevent an organization’s employees from sharing valuable company information or sensitive data—whether unwittingly or with ill intent—outside the network. DLP technologies can prevent actions that could potentially expose data to bad actors outside the networking environment, such as uploading and downloading files, forwarding messages, or printing.
- Firewalls.
- Firewalls are another common element of a network security model. They essentially function as a gatekeeper between a network and the wider internet. Firewalls filter incoming and, in some cases, outgoing traffic by comparing data packets against predefined rules and policies, thereby preventing threats from accessing the network.
etc
12. Write short notes on any two:[3.5+3.5]
a) Cyber law b) Normalization
ans:-
a)Cyber law:-
his law is commonly known as the law of the internet. It governs the legal issues of computers, Internet, data, software, computer networks and so on. These terms of legal issues are collectively known as cyberspace. In other words, it is a type of law which rules on the Internet to prevent Internet related crime.
Cyber law is a new and quickly developing area of the law that pertains to persons and companies participating in e-commerce development, online business formation, electronic copyright, web image trademarks, software and data licenses, online financial transactions, interactive media, domain name disputes, computer software and hardware, web privacy, software development and cybercrime which includes, credit card fraud, hacking, software piracy, electronic stalking and other computer related offenses.
b) Normalization:-
Some of the major benefits include the following :
- Greater overall database organization
- Reduction of redundant data
- Data consistency within the database
- A much more flexible database design
- A better handle on database security
- Fewer indexes per table ensures faster maintenance tasks (index rebuilds).
sources(with thanks):-
anydifferencebetween.com
sciencedaily.com
itstillworks.com
n-able.com
Merit Casino | New Orleans | USA | | 30 Second Chance
ReplyDeleteFeatures 바카라 추천 사이트 · Promotions · 우리 카지노 더킹 Promotions 카지노 사이트 주소 · Cash 바카라 사이트 주소 Offers emasgaraj.com · Promotions
Blackjack Casino Review 2021 - $20 Free + $5000 Bonus
ReplyDeleteBlackjack Casino is 오바마 카지노 가입 쿠폰 a blackjack-themed online casino offering blackjack, The site is 해븐 카지노 also bet analysis available on the web, including many 램 슬롯 different 양방 배팅